Resolver Integrales Dobles Rectangulares
- Gato Ingeniero
- 1 ene 2021
- 3 Min. de lectura
Actualizado: 6 ene 2022
-> Tiene su menú interactivo, aconsejo ver mas dentro del programa.
-> Mantiene una precisión comprobada de 9 decimas.
-> Presenta 3 métodos para resolver.
-> Trabaja las ecuaciones en funciones independientes.
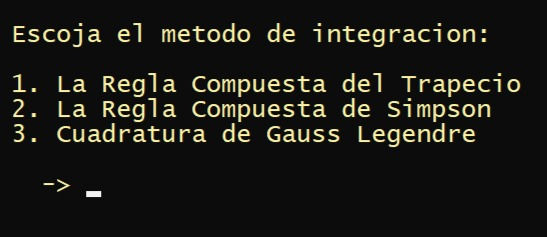
Acá les dejo el código:
///INTEGRALES DOBLES
#include <iostream>
#include <math.h>
#include <stdio.h>
#include <stdlib.h>
#include <iomanip>
using namespace std;
///---------------------------------------------------------------------------------------
struct Vect
{
double v[100];
};
///---------------------------------------------------------------------------------------
double pi=3.14159265 , e=2.71828183;
///---------------------------------------------------------------------------------------
void paso_inicial(double &h1,double &h2,double &n1,double &n2,double &c,double &d,double &a,double &b)
{
double aux5,aux6,aux1,aux2,aux3,aux4;
printf("\n ******DEVELOPED BY ALEXIS HUGO SEGALES RAMOSETH******\n");
cout<<"\n MODELO DE LA INTEGRAL: I[I F(xy) dx] dy";
cout<<"\n Limites de la integral EXTERNA: ";
cout<<"\n c= "; cin>>aux1; cout<<" d= "; cin>>aux2;
cout<<"\n # Intervalos: "; cin>>aux3;
c=aux1; d=aux2; n1=aux3;
h1=(double) (d-c)/n1;
cout<<"\n Limites de la integral INTERNA: ";
cout<<"\n a= "; cin>>aux4; cout<<" b= "; cin>>aux5;
cout<<"\n # Intervalos: "; cin>>aux6;
a=aux4; b=aux5; n2=aux6;
h2=(double) (b-a)/n2;
}
///---------------------------------------------------------------------------------------
void fun_trapecio(double &x,double &y,double &f)
{
f = (double) ((x*pow(e,y))+(y*pow(e,x))) / (pow(e,x)+pow(e,y));
}
///---------------------------------------------------------------------------------------
void metTrapecio()
{
Vect y,x,fx,S;
double suma2=0,suma,a,b,c,d,n1,n2,h1,h2,I,p2=0,p3=0;
paso_inicial(h1,h2,n1,n2,c,d,a,b);
system("cls");
for(int i=0 ; i<=n1 ; i++)
{
suma=0;
printf("\n %d. Integral Interna: ",i+1);
y.v[i]=(double) c + (i*h1);
printf("\n Desde a= %.10lf Hasta b= %.10lf Con paso h= %.10lf \n",a,b,h2);
for(int j=0 ; j<=n2 ; j++)
{
x.v[j]=(double) a + (j*h2);
fun_trapecio(x.v[j],y.v[i],fx.v[j]);
printf("\n x[%d]= %.10lf | Fx[%d]= %.10lf ",j,x.v[j],j,fx.v[j]);
if(j==0 || j==n2) p2+=fx.v[j];
}
for(int k=1 ; k<n2 ; k++)
{
suma=(double) suma+fx.v[k];
}
//printf("\n\n El valor de las sumas intermedias es : %.10lf ",suma);
S.v[i]=(float) (h2/2)*(p2+(2*suma));
if(i==0 || i==n1) p3+=S.v[i];
printf("\n");
}
printf("\n\n Los Valores de las Integrales Internas son: ");
for(int l=0 ; l<=n1 ; l++)
{
printf("\n %d. %.10lf ",l+1,S.v[l]);
}
for(int k=1 ; k<n1 ; k++)
{
suma2=(double) suma2+S.v[k];
}
I=(float) (h1/2)*(p3+(2*suma2));
printf("\n\n EL VALOR DE LA INTEGRAL DOBLE ES: %.10lf\n_",I);
}
///---------------------------------------------------------------------------------------
void fun_simpson(double &x,double &y,double &f)
{
f = (double) (x+y)/(cos(x)+cos(y));
}
///---------------------------------------------------------------------------------------
void metSimpson()
{
Vect y,x,fx,S;
double a,b,c,d,n1,n2,h1,h2,I,p1=0,p2=0,spa,sim,sp=0,si=0;
paso_inicial(h1,h2,n1,n2,c,d,a,b);
system("cls");
for(int i=0 ; i<=n1 ; i++)
{
sp=0,si=0;
printf("\n %d. Integral Interna: ",i+1);
y.v[i]=(double) c + (i*h1);
printf("\n Desde a= %.10lf Hasta b= %.10lf Con paso h= %.10lf \n",a,b,h2);
for(int j=0 ; j<=n2 ; j++)
{
x.v[j]=(double) a + (j*h2);
fun_simpson(x.v[j],y.v[i],fx.v[j]);
printf("\n x[%d]= %.10lf | Fx[%d]= %.10lf ",j,x.v[j],j,fx.v[j]);
if(j==0 || j==n2) p1+=fx.v[j];
}
for(int k=1 ; k<n2 ; k++)
{
if(k%2==0) sp=(double) sp+fx.v[k];
else si=(double) si+fx.v[k];
}
//printf("\n El valor de las sumas pares es : %.10f ",sp);
//printf("\n El valor de las sumas impares es: %.10f ",si);
S.v[i]=(float) (h2/3)*(p1+(4*si)+(2*sp));
//printf("\n El valor de la %d. Integral es: %.10lf",i+1,S.v[i]);
printf("\n");
}
printf("\n Los Valores de las Integrales Internas son: \n");
for(int l=0 ; l<=n1 ; l++)
{
printf(" %d. %.10lf ",l+1,S.v[l]);
if(l==0 || l==n1) p2+=S.v[l];
}
for(int k=1 ; k<n1 ; k++)
{
if(k%2==0)
spa=(double) spa+S.v[k];
else
sim=(double) sim+S.v[k];
}
I=(double) (h1/3)*(p2+(4*sim)+(2*spa));
printf("\n\n EL VALOR DE LA INTEGRAL DOBLE ES: %.10lf",I);
}
///---------------------------------------------------------------------------------------
void Lamb_Absc(int valor,Vect &abscisas,Vect &lambdas)
{
if(valor==2)
{
abscisas.v[0]=(double) -1/sqrt(3);
abscisas.v[1]=(double) 1/sqrt(3);
lambdas.v[0]=1;
lambdas.v[1]=1;
}
if(valor==3)
{
abscisas.v[0]=(double) -sqrt(0.6);
abscisas.v[1]= 0;
abscisas.v[2]=(double) sqrt(0.6);
lambdas.v[0]=(double) 5/9;
lambdas.v[1]=(double) 8/9;
lambdas.v[2]=(double) 5/9;
}
if(valor==4)
{
abscisas.v[0]=(double) -0.861136311594053;
abscisas.v[1]=(double) -0.339981043584856;
abscisas.v[2]=(double) 0.339981043584856;
abscisas.v[3]=(double) 0.861136311594053;
lambdas.v[0]=(double) 0.347854845137454;
lambdas.v[1]=(double) 0.652145154864546;
lambdas.v[2]=(double) 0.652145154864546;
lambdas.v[3]=(double) 0.347854845137454;
}
if(valor==5)
{
abscisas.v[0]=(double) -0.906179845938664;
abscisas.v[1]=(double) -0.538469310105683;
abscisas.v[2]= 0;
abscisas.v[3]=(double) 0.538469310105683;
abscisas.v[4]=(double) 0.906179845938664;
lambdas.v[0]=(double) 0.236926885056189;
lambdas.v[1]=(double) 0.478628670499366;
lambdas.v[2]=(double) (128/225);
lambdas.v[3]=(double) 0.478628670499366;
lambdas.v[4]=(double) 0.236926885056189;
}
if(valor==6)
{
abscisas.v[0]=(double) -0.932469514203152;
abscisas.v[1]=(double) -0.661209386466265;
abscisas.v[2]=(double) -0.238619186083197;
abscisas.v[3]=(double) 0.238619186083197;
abscisas.v[4]=(double) 0.661209386466265;
abscisas.v[5]=(double) 0.932469514203152;
lambdas.v[0]=(double) 0.171324492379171;
lambdas.v[1]=(double) 0.360761573048139;
lambdas.v[2]=(double) 0.467913934572691;
lambdas.v[3]=(double) 0.467913934572691;
lambdas.v[4]=(double) 0.360761573048139;
lambdas.v[5]=(double) 0.171324492379171;
}
}
///---------------------------------------------------------------------------------------
void fun_gausslegendre(double &x,double &y,double &f)
{
f = (double) sqrt(pow(e,-(cos(x))) + pow(e,-(sin(y))));
}
///---------------------------------------------------------------------------------------
void metGaussLegendre()
{
Vect y,x,fx,S,abscisas,lambdas,lamfun,Suma;
double a,b,c,d,n1,n2,h1,h2,I,AA,BB,AAAA,BBBB,s,suma=0;
paso_inicial(h1,h2,n1,n2,c,d,a,b);
AA=(double) (d-c)/2;
BB=(double) (d+c)/2;
AAAA=(double) (b-a)/2;
BBBB=(double) (b+a)/2;
system("cls");
if(n1==2) Lamb_Absc(n1,abscisas,lambdas);
if(n1==3) Lamb_Absc(n1,abscisas,lambdas);
if(n1==4) Lamb_Absc(n1,abscisas,lambdas);
if(n1==5) Lamb_Absc(n1,abscisas,lambdas);
if(n1==6) Lamb_Absc(n1,abscisas,lambdas);
for(int i=0 ; i<n1 ; i++)
{
printf(" %d. INTEGRAL INTERNA: ",i+1);
Lamb_Absc(n1,abscisas,lambdas);
y.v[i]=(double) (AA*abscisas.v[i])+BB;
printf("\n CON EL VALOR DE y[%d]= %.10lf ",i+1,y.v[i]);
printf(" DESDE a= %.10lf HASTA b= %.10lf \n",a,b);
printf("\n i t[i] x[i] fx[i] lambda[i] lambda[i]*fx[i] \n");
s=0;
for(int j=0 ; j<n2 ; j++)
{
x.v[j]=(double) (AAAA*abscisas.v[j])+BBBB;
fun_gausslegendre(x.v[j],y.v[i],fx.v[j]);
lamfun.v[j]=(double) (lambdas.v[j]*fx.v[j]);
printf("\n %d %.10f %.10f %.10f %.10f %.10f ",j+1,abscisas.v[j],x.v[j],fx.v[j],lambdas.v[j],lamfun.v[j]);
s=s+lamfun.v[j];
}
for(int k=1 ; k<n1 ; k++)
{
S.v[k]=(double) s*BBBB;
printf("\n LA SUMA DE lambda*F[x] ES= %.10f \n",S.v[k]);
Suma.v[i]=S.v[k];
}
}
for(int k=0 ; k<n1 ; k++)
{
suma=(double) suma+Suma.v[k];
}
I=AA*suma;
printf("\n\n EL VALOR DE LA INTEGRAL DOBLE ES: %.10lf \n",I);
}
///---------------------------------------------------------------------------------------
void menumetodos()
{
int opcion;
printf("\n\n Escoja el metodo de integracion:\n");
cout<<"\n 1. La Regla Compuesta del Trapecio";
cout<<"\n 2. La Regla Compuesta de Simpson";
cout<<"\n 3. Cuadratura de Gauss Legendre";
cout<<"\n\n -> ";cin>>opcion;
if(opcion==1)
{
system("cls");
metTrapecio();
}
if(opcion==2)
{
system("cls");
metSimpson();
}
if(opcion==3)
{
system("cls");
metGaussLegendre();
}
}
///---------------------------------------------------------------------------------------
void limppant()
{
printf("\n Desea iniciar de nuevo \n 1)SI \n 2)NO ");
int mess; cin>>mess;
if(mess==1)
{
system("cls");
system("rst");
menumetodos();
limppant();
}
if(mess==2)
{
system("close");
}
}
///---------------------------------------------------------------------------------------
int main()
{
menumetodos();
limppant();
return 0;
}
Comments